Getting Started
The Airship Windows SDK supports Windows and Windows Phone devices, including Windows 10.
Apps targeting Windows Phone 8.1 and higher should use the Universal Library, which is compatible with Windows Desktop and Windows Phone via the WNS push service.
Windows devices provide a unique notification user experience with live tiles, a set of app icon assets that can be customized to reflect your app’s branding.
Windows devices also support toast notifications, the familiar non-modal notification element often used to display a short, auto-expiring message in many Android and desktop applications.
For details on the Windows notification user experience, see the following resources from Microsoft:
Supported Features
Airship provides Support for Live Tiles and Toast notifications. We support Tiles at the API Level only, and Toast both at the API level and in the dashboard.
We support Toast Templates at the API level as well.
See Windows Push for examples of tiles and toast notifications.
Key Terminology
- APID
- Sometimes also described as a “Push ID”, and APID is an app-level device identifier that can be used to target specific devices with push notifications. All Windows apps using the Airship library are assigned an APID, which takes the form of a UUID string, such as
e3d35643-26d3-4b0e-c632-959291744a02
. - App Key and Secret
- All applications registered with Airship have an associated key, secret and master secret, which are strings used in authentication with the Airship REST API. The app key is effectively an identifier. The app secret is used in authentication on the client side during registration.
- Master Secret
- There is also another secret, known as the master secret, that is used in authentication for REST API actions such as sending push notifications. This is the only one of these that should be kept truly secret, because with this, anyone could could send push notifications on your behalf.
Always keep the master secret safe, and be sure never to expose it in your application code.
To obtain these strings, create a new application on the Airship dashboard or log in to your existing app. In the sidebar on the left side of the screen, click Details. In the resulting window, you should find the key and secret at the top of the list. Here is an overview of the steps:
Configure Services
- Secure your Windows Push Notification Services (WNS) service API keys from Microsoft.
- For Windows 8+ and Windows Phone 8.1+ (WNS) see How to authenticate with the Windows Push Notification Service (WNS) (Windows Runtime apps) .
- Download the latest Airship SDK here .
- Read our client documentation. (Below)
- Integrate our client library into your development application, per the documentation.
- Configure the Windows service in the DashboardThe Airship web interface located at go.airship.com or go.airship.eu..
- Deploy your application to the Windows Store.
Universal Library
This document provides reference information for implementing a client for a Windows desktop, phone or tablet (e.g. Surface tablet) device.
Set Up Your Application in the Windows Store
In order to be able to receive push notifications, you must set up your application in the Windows Store. If your app is not already registered with the Windows Store, please review How to authenticate with the Windows Push Notification Service (WNS) on MSDN, and follow the instructions before continuing.
Once your app is registered with the Windows Store, and you have logged in as a developer, navigate to: App »» Advanced Features »» Push notifications and Live Connect services info. If you are submitting a Windows Phone 8.1 app, log in to the Windows Phone Dev Center, select your app, then navigate to the Details tab and look for the WNS heading. You will need to make note of the following pieces of information:
- CN and Identity Name: Under the “Identifying your app” link on the sidebar.
- SID and Client Secret: Under the “Authenticating your service” link on the sidebar
The CN and Identity Name must now be added to your package manifest. You can set them manually on the “Packaging” tab, or you can let Visual Studio automatically associate your project with the application in the Windows Store by right clicking on the project and selecting Store->Associate App with the Store.
Set Up Your Project with Airship
It is customary on Airship to create two separate projects in the DashboardThe Airship web interface located at go.airship.com or go.airship.eu., one for development and one for production. If you haven’t already, perform this step now. In order to keep them distinct, it is recommended to choose names that reflect their purpose in the development lifecycle, e.g. “My Development App” vs. “My Production App”. Repeat the process below for each app.
You will need your Windows Push Notification Services (WNS):
- Package security identifier (SID)
- Secret key
You can find these in the Partner Center ; follow the instructions on the App Management - WNS/MPNS page.
- Go to Settings » Channels » Mobile Apps » Windows.
- Enter your SID and secret key.
- Click Save.
Client Library
Add UrbanAirship.winmd
To add the UrbanAirship runtime component to your application:
- Unzip the distribution file (urban-airship-windows-
.zip) - Copy the
UrbanAirshipLibrary
folder to a location in your project path. - Add a reference to
UrbanAirship.winmd
.
Initialize the Airship Library
The library must be initialized with your Airship application key and application secret. If you have created separate applications for development and production, as is recommended above, this is a good opportunity to specify the key/secret pair for each mode.
Add airshipconfig.xml
to your project
airshipconfig.xml
<?xml version="1.0" encoding="utf-8" ?>
<AirshipConfig>
<!-- NOTE: These elements must be in alphabetical order. -->
<DevelopmentAppKey>Development Key</DevelopmentAppKey>
<DevelopmentAppSecret>Development Secret</DevelopmentAppSecret>
<DevelopmentLogLevel>Verbose</DevelopmentLogLevel>
<InProduction>false</InProduction>
<ProductionAppKey>Production App Key</ProductionAppKey>
<ProductionAppSecret>Production App Secret</ProductionAppSecret>
<ProductionLogLevel>Error</ProductionLogLevel>
</AirshipConfig>
This file contains all the settings your app needs to register and receive push notifications. These settings can also be defined programmatically; see the integration section for details.
Initialize the Airship Library
App.xaml.cs
using UrbanAirship;
using UrbanAirship.Push;
//...
/// <summary>
/// Initializes the singleton application object. This is the first line of authored code
/// executed, and as such is the logical equivalent of main() or WinMain().
/// </summary>
public App()
{
this.InitializeComponent();
this.Suspending += OnSuspending;
// Initialize and register UA event listeners
// See example implementations below
this.registrationListener = new RegistrationEventListener();
this.pushReceivedListener = new PushReceivedEventListener();
this.pushActivatedListener = new PushActivatedEventListener();
}
/// <summary>
/// Invoked when the application is launched normally by the end user. Other entry points
/// will be used when the application is launched to open a specific file, to display
/// search results, and so forth.
/// </summary>
/// <param name="args">Details about the launch request and process.</param>
protected override void OnLaunched(LaunchActivatedEventArgs args)
{
Logger.Debug("Launched with: " + args.Arguments);
Frame rootFrame = Window.Current.Content as Frame;
// Do not repeat app initialization when the Window already has content,
// just ensure that the window is active
if (rootFrame == null)
{
// Create a Frame to act as the navigation context and navigate to the first page
rootFrame = new Frame();
if (args.PreviousExecutionState == ApplicationExecutionState.Terminated)
{
//TODO: Load state from previously suspended application
}
// Place the frame in the current Window
Window.Current.Content = rootFrame;
}
if (rootFrame.Content == null)
{
// When the navigation stack isn't restored navigate to the first page,
// configuring the new page by passing required information as a navigation
// parameter
if (!rootFrame.Navigate(typeof(MainPage), args.Arguments))
{
throw new Exception("Failed to create initial page");
}
}
// Ensure the current window is active
Window.Current.Activate();
//Initialize and setup UA library
var config = AirshipConfig.ReadConfig("airshipconfig.xml");
UAirship.TakeOff(config);
config.DebugLog();
PushManager.Shared.EnabledNotificationTypes = NotificationType.Toast;
}
Configure Keys and Secrets in Code
These configuration values can also be set programatically:
AirshipConfig config = new AirshipConfig();
config.DevelopmentAppKey = "DevelopmentAppKey";
config.DevelopmentAppSecret = "DevelopmentAppSecret";
config.ProdutionAppKey = "ProductionAppKey";
config.productionAppSecret = "ProductionAppSecret";
#if DEBUG
config.InProduction = false;
#else
config.InProduction = true;
#endif
UAirship.TakeOff(config);
Manifest Permissions
To enable push notifications in your Windows Store app, you will need to add several items to your app’s manifest (Package.appxmanifest). The items below are organized by tab
Application UI
On the “Application UI” tab, make sure that “Toast capable” is checked.
Capabilities
Make sure that “Internet” is checked.
Declarations
Push Channels have an expiration date and must be renewed periodically. To ensure that the channel is renewed even if the user hasn’t launched the app recently, our library schedules a background task that will perform the renewal in the background.
- Open the manifest (
Package.appxmanifest
). - On the Declarations tab, Add a Background Task.
- Set the task type to System event.
- Set the Entry Point to
UrbanAirship.Push.WNSChannelRenewalTask
.
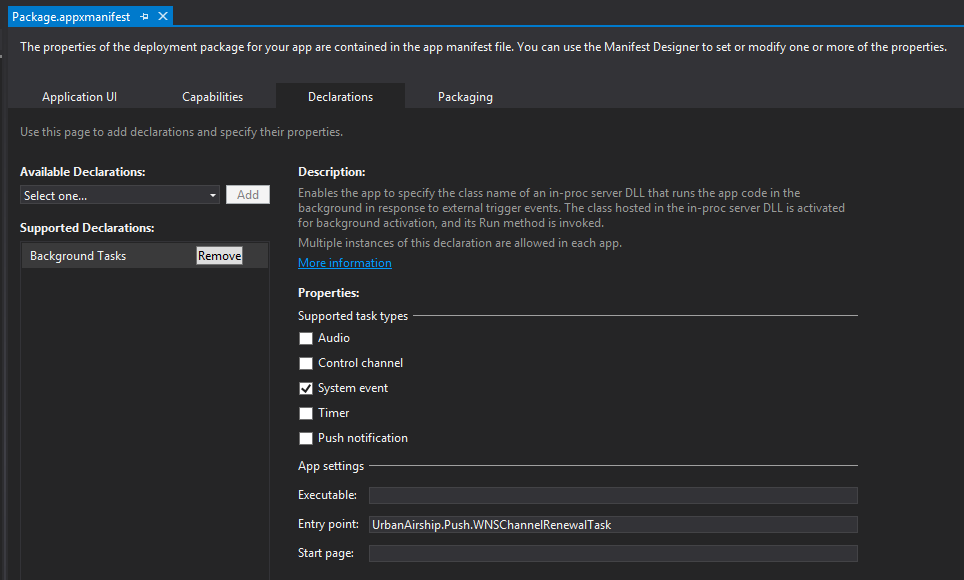
Categories