Analytics and Reporting
Learn how to use Airship analytics.
The Airship SDK stores batches of events and uploads them periodically in the background. We’ve taken great care to ensure that the database won’t grow beyond a small fixed size, so extended periods of lost connectivity are nothing to worry about. The event upload thread is woken up when new events are triggered and goes to sleep when there are no more events to process, so the impact on battery life is negligible.
For information about data collection and privacy, see Data Collection.
Custom Events
Custom events let you track user activities and key conversions in your application and tie them back to corresponding push messaging campaigns. They require enabling analytics for your app. If disabled, any event that is added to analytics will be ignored.
Events are distinct from tags, which describe the condition of a user, such as a stated preference or information about their app version. Tags are static and can represent something like “opted in for sales alerts” (sales_opt_in
). Custom events are dynamic and can represent something like “read more than five sports articles” (read_>5_sports_articles
), and additional events occur when a user reads more articles. The benefit of using custom events is that they follow a user behaviors. For a more detailed explanation, see the Custom Events guide.
Custom events serve two purposes: messaging and measurement. The best way to get started with custom events is to look at each page or screen of your digital experience and note every action a user can take. Do you want to measure how often a user does that specific action, such as “viewed content” or “added to cart”? Would you want to message a user after they completed that task for the first time or after the third time?
Make each of the user actions custom events. You’ll then be able to trigger messaging after the event occurs. You can also measure the effectiveness or use of these features.
CustomEvent.newBuilder("event_name")
.setEventValue(123.12)
.addProperty("my_custom_property", "some custom value")
.addProperty("is_neat", true)
.addProperty(
"any_json", JsonMap.newBuilder()
.put("foo", "bar")
.build()
)
.build()
.track()
CustomEvent.newBuilder("event_name")
.setEventValue(123.12)
.addProperty("my_custom_property", "some custom value")
.addProperty("is_neat", true)
.addProperty("any_json", JsonMap.newBuilder()
.put("foo", "bar")
.build())
.build()
.track();
let event = CustomEvent(name: "event_name", value: 123.12)
event.properties = [
"my_custom_property": "some custom value",
"is_neat": true,
"any_json": [
"foo": "bar"
]
]
event.track()
UACustomEvent *event = [UACustomEvent eventWithName:@"event_name" value:@123.12];
event.properties = @{
@"my_custom_property": @"some custom value",
@"is_neat": @YES,
@"any_json": @{
@"foo": @"bar"
}
};
[event track];
var customEvent = new UACustomEvent("event_name", 123.12);
customEvent.addProperty("my_custom_property", "some custom value");
customEvent.addProperty("is_neat", true);
customEvent.addProperty("any_json", {
"foo": "bar"
});
await Airship.analytics.addCustomEvent(customEvent);
CustomEvent event = CustomEvent(
name: "event_name",
value: 123.12,
properties: {
"my_custom_property": "some custom value",
"is_neat": true,
"any_json": {
"foo": "bar"
}
}
);
Airship.analytics.recordCustomEvent(event);
let event = {
eventName: "event_name",
eventValue": 123.12,
properties: {
"my_custom_property": "some custom value",
"is_neat": true,
"any_json": {
"foo": "bar"
}
}
}
Airship.analytics.addCustomEvent(event)
let event = {
eventName: "event_name",
eventValue": 123.12,
properties: {
"my_custom_property": "some custom value",
"is_neat": true,
"any_json": {
"foo": "bar"
}
}
}
await Airship.analytics.addCustomEvent(event)
using AirshipDotNet;
CustomEvent customEvent = new CustomEvent();
customEvent.EventName = "event_name";
customEvent.EventValue = 123.45;
customEvent.AddProperty("my_custom_property", "some custom value");
customEvent.AddProperty("is_neat", true);
Airship.Instance.AddCustomEvent(customEvent);
using UrbanAirship.NETStandard;
CustomEvent customEvent = new CustomEvent();
customEvent.EventName = "event_name";
customEvent.EventValue = 123.45;
customEvent.AddProperty("my_custom_property", "some custom value");
customEvent.AddProperty("is_neat", true);
Airship.Instance.AddCustomEvent(customEvent);
var event = Airship.analytics.newEvent("event_name")
event.eventValue = 123.45
event.eventProperties = {
is_neat: true,
some_custom_: 124.49,
my_custom_property: "some custom value",
any_json: {
foo: "bar"
}
}
event.track()
CustomEvent customEvent = new CustomEvent();
customEvent.EventName = "event_name";
customEvent.EventValue = 123.45;
customEvent.AddProperty("my_custom_property", "some custom value");
customEvent.AddProperty("is_neat", true);
UAirship.Shared.AddCustomEvent(customEvent);
Testing Custom Events
Once you define your custom events and use cases, you can start messaging users based on these custom events and behaviors within the app. See:
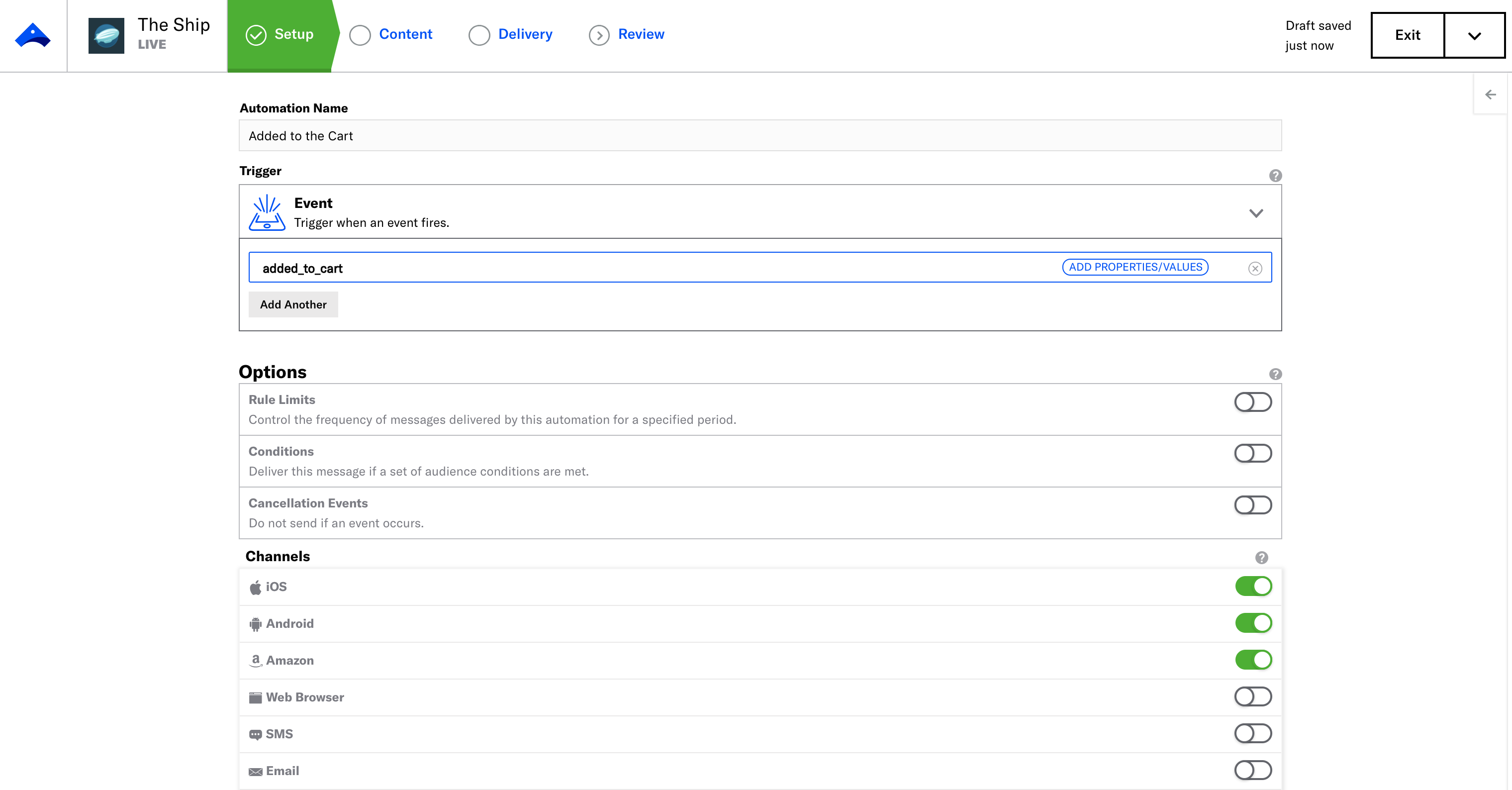
You can also see custom events in Performance AnalyticsA customizable marketing intelligence tool that provides access to reports and graphs based on engagement data..
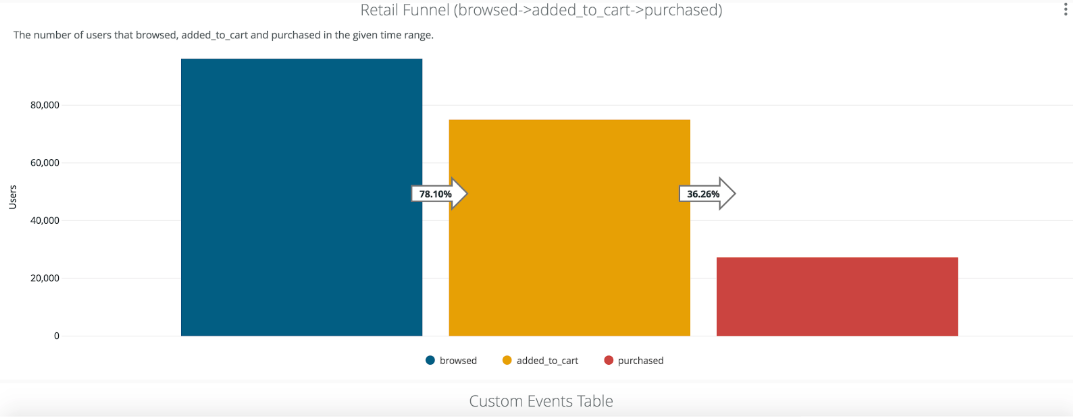
Custom Identifiers
A custom identifier associates an external identifier with a Channel IDAn Airship-specific unique identifier used to address a channel instance, e.g., a smartphone, web browser, email address.. They are visible in Real-Time Data StreamingA service that delivers engagement events in real time via the Data Streaming API or an Airship partner integration.. We recommend adding any IDs that you may want to be visible in your event stream. You can assign up to 20 custom identifiers to a device. Unlike other identifiers (e.g., tags), you cannot use custom identifiers to target your users.
Setting custom identifiers
UAirship.shared().analytics
.editAssociatedIdentifiers()
.addIdentifier("key", "value")
.apply()
UAirship.shared().getAnalytics()
.editAssociatedIdentifiers()
.addIdentifier("key", "value")
.apply();
let identifiers = Airship.analytics.currentAssociatedDeviceIdentifiers()
identifiers.set(identifier: "value", key:"key")
Airship.analytics.associateDeviceIdentifiers(identifiers)
UAAssociatedIdentifiers *identifiers = [UAirship.analytics currentAssociatedDeviceIdentifiers];
identifiers.setIdentifier("value", forKey:"key")
[UAirship.analytics associateDeviceIdentifiers:identifiers];
await Airship.analytics.associateIdentifier("key", "value");
Airship.analytics.associateIdentifier("key", "value");
Airship.analytics.setAssociatedIdentifier("key", "value")
await Airship.analytics.associateIdentifier("key", "value")
using AirshipDotNet;
Airship.Instance.AssociateIdentifier("key", "value");
using UrbanAirship.NETStandard;
Airship.Instance.AssociateIdentifier("key", "value");
Airship.analytics.editAssociatedIdentifiers()
.setIdentifier("key", "value")
.apply()
UAirship.Shared.AssociateIdentifier("key", "value");
Screen Tracking
The Airship SDK gives you the ability to track which screens a user views within the application, how long a user stayed on each screen, and also includes the user’s previous screen. These events then come through Real-Time Data StreamingA service that delivers engagement events in real time via the Data Streaming API or an Airship partner integration., allowing you to see the path that a user took through the application, or trigger actions based on a user visiting a particular area of the application.
Track a screen
UAirship.shared().analytics.trackScreen("MainScreen")
UAirship.shared().getAnalytics().trackScreen("MainScreen");
Airship.analytics.trackScreen("MainScreen")
[UAirship.analytics trackScreen:@"MainScreen"];
await Airship.analytics.trackScreen("MainScreen");
Airship.analytics.trackScreen("MainScreen");
Airship.analytics.trackScreen("MainScreen")
await Airship.analytics.trackScreen("MainScreen")
using AirshipDotNet;
Airship.Instance.TrackScreen("MainScreen");
using UrbanAirship.NETStandard;
Airship.Instance.TrackScreen("MainScreen");
Airship.analytics.trackScreen("MainScreen")
UAirship.Shared.TrackScreen("MainScreen")
Categories